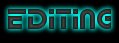
Editing a Mission Briefing
by Ed Benowitz and Phillip Ogden
This section discusses the new briefing features, using both a .xml
file and a .lua file, a new feature in 1.5. You can still create old mission briefings.
Basic Concepts
A mission briefing appears before a given mission. It is used for
storytelling, to get the player prepared for the mission. In
every mission briefing, the camera movement is already predetermined.
All you have to do is provide locations for nav points, ships,
and some accompanying story text, and the mission briefing takes care
of the rest.
Mission Briefing File
The mission briefing file must be named correctly. The briefing
filename is the same as the mission filename, except that the briefing
file name ends in .briefing.xml.
For example, if the mission is named mission_a1.mission.xml, then the briefing must be named mission_a1.briefing.xml.
First, it is easist just to copy over an already created briefing file,
but here is a basic form for it that will make things a little easier
to change out.
Okay, first you have the initial lines.
<?xml version="1.0" encoding="UTF-8"?>
<briefing xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="briefing.xsd">
Navpoints
We add nav points. A briefing can have
any number of Nav Points. Nav points appear as green circles.
The navpoints element acts as a container for each individual nav point.
<navpoints>
<navpoint radius="200.0">
<location>
<point x="400.0" y="2.66" z="500.0"/>
</location>
</navpoint>
</navpoints>
Now the first thing in a nav is it's radius or size. It is best to have
capship navs with a radius of 200 and a radius of 100 for empty or
fighter navs. Next, choose a location for the nav point.
The center of the map is at 0, 0, 0. Keep in mind the
radius of this and other nav points as you choose your location, you
don't want nav points to overlap.
Optionally, you can also specify a nav point name that will be displayed in the briefing.
<navpoint radius="200.0" name="Nav 2">
Also, to reference the nav point in scripts, give the nav point a script is
<navpoint radius="200.0" name="Nav 2" scriptid="$nav2">
Ships
Ships can either travel with the player through the navs, or they can
stay at a fixed position at a nav. When the ship moves with the
player, we call this autopiloting.
If the ship is autopiloting, when we specify the location, the location
is always relative to the player's position. y is up, x is to the
right, and z is forward. For example, to place a wingman to the
right of a player, we would specify (20, 0, 0).
However if a ship is not autopiloting, the location takes on a
completely different meaning. In this case, the location is
relative to the center of the nav point. y is up, x
is to the right, and z is forward.
It will
take a little trial and error, though, to get the location right.
The player only has the
mesh already defined at the top for the xml file, but for your wingmen, they will have
to be defined in the nav they start in. Here is your wingman:
<ship name="piranha.mesh.bin" alignment="friendly" capship="false" scriptid="$playership">
<location>
<point x="20.0" y="0.0" z="0.0"/>
</location>
</ship>
let's look at each field
name: nave is the name of the mesh file of the ship. These files must end in .mesh.bin or .obj
alignment: Used to control the color of the ship. Can be either friendly or enemy.
capship: Used to control the color of a ship. Set to false for fighters.
scriptid: Used to refer to the ship within the briefing script.
location: specifies the location of the ship, in this case relative to the nav center.
Capships
For capships, you may have to add in a scale to decrease the size of the mesh in the briefing. Here is the Midway:
<ship name="midway.mesh.bin" autopilot="false" alignment="friendly" capship="true" scale=".05">
<location>
<point x="60.0" y="0.0" z="0.0"/>
</location>
</ship>
Conclusion
With this information, copy over mission_a1.briefing.xml, and use
it as a template for creating your own mission briefings.
Briefing Scripting
Note that only the below functions can be used for briefing scripting.
Please see mission_a1.briefing.lua for an example mission
briefing.
The briefing script file needs to be named specially. If your
mission file is mission_a1.mission.xml, then your briefing file must be
named mission_a1.briefing.lua.
The first line of a briefing script should always be
dofile "flightcommander_briefing.lua"
There are two functions which you must implement in your briefing script file.
function startup()
is called to setup your script. In this function, you can setup initial positions, for example.
Then your
function advance()
will be called 4 times a second. In this function, check the
time. Use the time and your own variables to determine what to
do. Move ships and the cameras around, play sounds, and set the
overlay text, for example.
Briefing Script Reference
Briefing_playSound(string filename)
Plays a sound during a briefing. Filename is either a wav file or an
mp3 file. Only one sound can play at a time. This is
typically used for a briefing voice over.
since 1.5
float x, float y,
float z BriefingNav_getLocation(string scriptid)
This function returns three values, the location of the nav point.
This is the location in world space of the nav point's center. If the nav cannot be found, 3 nil values are
returned.
Example:
x, y, z = BriefingNav_getLocation("midway");
since 1.5
float x, float y,
float z BriefingShip_getLocation(string scriptid)
This function returns three values, the location of the ship.
This is the location in world space of the ship's center of
rotation. If the ship cannot be found, 3 nil values are
returned.
Example:
x, y, z = BriefingShip_getLocation("midway");
since 1.5
BriefingShip_setColor(string scriptid, float r, float g, float b)
Changes the color of the briefing ship. Each of r, g, b, ranges from 0.0 to 1.0
since 1.5
BriefingShip_setHeading(string scriptid, float x, float y, float z)
A unit vector in the direction the ship should be heading.
since 1.5
BriefingShip_setHeadingFixedNavs(string shipid, string nav1id, string nav2id)
Sets the heading on the ship. The heading is set as if the ship were moving from nav1 to nav 2.
shipid is the script id of the ship
nav1id is the script id of nav1
nav2id is the script id of nav 2
since 1.5
BriefingShip_setHeadingInterpNavs(string shipid, string nav1id, string nav2id, string nav3id, int timems)
Rotates the heading of a ship over time. The heading is set as if
the ship just finished moving from nav1 to nav 2, and is turning
so that it can move from nav2 to nav 3.
shipid is the script id of the ship
nav1id is the script id of nav1
nav2id is the script id of nav 2
nav3id is the script id of nav 3
timems is the time it takes to change headings, in milliseconds.
since 1.5
BriefingShip_setHeadingInterpolated(string scriptid, float fromx, float fromy, float fromz,
float tox, float toy, float toz, int timems)
Interpolated a unit vector in the direction the ship should be heading.
The timems indicates how many milliseconds (ms) it takes to do
the move.
since 1.5
BriefingShip_setHeadingRelative(string myscriptid, string theirscriptid)
Forces the heading of the ship identified by myscriptid to be the same as the heading of another ship at all times.
since 1.5
BriefingShip_setLocation(string scriptid, float x, float y, float z)
This function sets the world space location of the ship.
since 1.5
BriefingShip_setLocationInterpolated(string scriptid, float fromx, float fromy, float fromz,
float tox, float toy, float toz, int timems)
This function sets the world space location of the ship. The ship
moves from the "from" location to the "to" location. The timems
indicates how many milliseconds (ms) it takes to do the move.
since 1.5
BriefingShip_setLocationInterpNavs(string shipid, string nav1, string nav2, int timems)
Moves the ship specified by shipid from nav1 to nav2. This happens over timems milliseconds
shipid is the script id of the ship
nav1id is the script id of nav1
nav2id is the script id of nav 2
since 1.5
BriefingShip_setLocationNav(string shipid, string navid)
Sets the ship at the center of the nav.
shipid is the script id of the ship
navid is the script id of the nav
since 1.5
BriefingShip_setLocationRelative(string myshipid, string theirshipid, float relativex, float relativey, float relativez)
Sets myshipid's location so that it moves with theirshipid. In
object space, myshipid's location is the same location as theishipid,
plus an offset of
(relativex, relativey, relativez).
since 1.5
BriefingShip_setVisible(string shipid, boolean visible);
By default briefing ships are visible, but by calling this with visible set to false, you can hid briefing ships from the user.
since 1.5
Camera_setLocationFixed(float
x, float y, float z)
Sets the camera's position. For use only
with CAMERA_SCRIPTABLE.
since 1.4
Camera_setLookAtFixed(float
x, float y, float z)
Sets the camera's look at. For use only with CAMERA_SCRIPTABLE.
since 1.4
Camera_setLocationInterpolated(float
fromx, float fromy, float fromz,
float
tox, float toy, float
toz,
int
timeinmilliseconds)
Moves the camera's position from the from location to the tolocation,
over a time period. The time it takes to do this is
timeinmilliseconds. For use only with CAMERA_SCRIPTABLE.
since 1.4
Camera_setLookAtInterpolated(float
fromx, float fromy, float fromz,
float
tox, float toy, float
toz,
int
timeinmilliseconds)
Moves the camera's look at from the from location to the tolocation,
over a time period. The time it takes to do this is
timeinmilliseconds. For use only with CAMERA_SCRIPTABLE.
since 1.4
Camera_setLocationRelative(string
shipid, float offsetx, float offsety, float offsetz)
Sets the camera's location relative to the position of the ship.
As the ship moves, the camera moves with it. For use only
with CAMERA_SCRIPTABLE. The offset is in world space, not
object space. This differs
from Camera_setLocationRelativeObject in that if the ship
rotates, the camera does NOT rotates along with it.
since 1.4
Camera_setLocationRelativeObject(string
shipid, float offsetx, float offsety, float offsetz)
Sets the camera's location relative to the position of the ship.
As the ship moves, the camera moves with it. For use only
with CAMERA_SCRIPTABLE. The offset is in object space, not
world space. This differs from Camera_setLocationRelative in that
if the ship rotates, the camera rotates along with it.
since 1.5
Camera_setLookAtRelative(string
shipid)
Sets the camera's look at the be the ship. As
the ship moves, the camera's look at moves with it. For use only
with CAMERA_SCRIPTABLE.
since 1.4
Camera_setLookAtInterpolatedShips(string
fromshipid, string toshipid, int timeinmilliseconds)
Interpolates the camera's lookat between the location of two
ships. The time it takes to do this is
timeinmilliseconds. For use only with CAMERA_SCRIPTABLE.
since 1.4
float hx, float hy, float hz computeheading(float p1x,float p1y, float p1z, float p2x, float p2y, float p2z)
Computer the heading (a unit vector) in the direction from point 1 to point 2.
(p1x, p1y, p1z) is point 1
(p2x, p2y, p2z) is point 2
(hx, hy, hz) is a unit heading vector, for use with one of the set heading functions.
since 1.5
int
Mission_getElapsedTime();
Returns the number of milliseconds since the start of the mission briefing.
Example:
print(Mission_getElapsedTime() );
Overlay_setText(string
newtext)
Sets the text that is displayed by the overlay.
since 1.4
TextToSpeech(string text, string wavfilename)
Uses a speech synthesizer to create a sound file. Text is
the text that will be spoken. wavfilename is the name of the
output file.
since 1.5